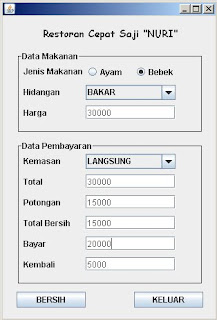
Ketentuan soal :
1. Hidangan
--------------------------------
AYAM BAKAR = Rp. 20.000,-
AYAM GULAI = Rp. 15.000,-
BEBEK BAKAR = Rp. 30.000,-
BEBEK GULAI = Rp. 25.000,-
--------------------------------
2. Kemasan
--------------------------------
PESAN = Diskon 10%
LANGSUNG = Diskon 50%
--------------------------------
Source Code :
---------------------------------------------------------------------------------------
package mykalkulator;
import javax.swing.JOptionPane;
/**
*
* @author aie_sendiri
*/
public class FRESTO extends javax.swing.JFrame {
/** Creates new form FRESTO */
public FRESTO() {
initComponents();
}
/** This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// Cara Pembuatan Method :
void hitung(){
if(chidangan.getSelectedItem().toString().equalsIgnoreCase("BAKAR") && rbtnayam.isSelected()){
tharga.setText("20000");
ckemasan.requestFocus();
}
else if(chidangan.getSelectedItem().toString().equalsIgnoreCase("BAKAR") && rbtnbebek.isSelected()){
tharga.setText("30000");
ckemasan.requestFocus();
}
else if(chidangan.getSelectedItem().toString().equalsIgnoreCase("GULAI") && rbtnayam.isSelected()){
tharga.setText("15000");
ckemasan.requestFocus();
}
else if(chidangan.getSelectedItem().toString().equalsIgnoreCase("GULAI") && rbtnbebek.isSelected()){
tharga.setText("25000");
ckemasan.requestFocus();
}
else{
tharga.setText("0");
rbtnayam.requestFocus();
}
}
---------------------------------------------------------------------------------------
1. Radio Button Ayam :
private void rbtnayamActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
// Pemanggilan method :
hitung();
}
2. Radio Button Bebek :
private void rbtnbebekActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
// Pemanggilan method :
hitung();
}
3. Combo Box Hidangan :
private void chidanganActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
// Pemanggilan method :
hitung();
}
4. Combo Box Kemasan :
private void ckemasanActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
if(ckemasan.getSelectedItem().toString().equalsIgnoreCase("PESAN")){
int total = Integer.parseInt(tharga.getText());
ttotal.setText(String.valueOf(total));
int pot = total * 10/100;
tpot.setText(String.valueOf(pot));
int totber = total - pot;
ttotber.setText(String.valueOf(totber));
tbayar.requestFocus();
}
else if(ckemasan.getSelectedItem().toString().equalsIgnoreCase("LANGSUNG")){
int total = Integer.parseInt(tharga.getText());
ttotal.setText(String.valueOf(total));
int pot = total * 50/100;
tpot.setText(String.valueOf(pot));
int totber = total - pot;
ttotber.setText(String.valueOf(totber));
tbayar.requestFocus();
}
else{
ttotal.setText("0");
tpot.setText("0");
ttotber.setText("0");
ckemasan.requestFocus();
}
}
5. Text Field Bayar :
private void tbayarActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
int totber = Integer.parseInt(ttotber.getText());
int bayar = Integer.parseInt(tbayar.getText());
if(bayar>=totber){
int kembali = bayar - totber;
tkembali.setText(String.valueOf(kembali));
}
else{
int validasi = JOptionPane.showConfirmDialog(null,"MAAF, Uang Bayar Kurang !!","Pemberitahuan",JOptionPane.OK_CANCEL_OPTION);
if( validasi==JOptionPane.OK_OPTION){
tbayar.setText("");
tkembali.setText("0");
tbayar.requestFocus();
}
else{
tbayar.setText("");
tkembali.setText("0");
tbayar.requestFocus();
}
}
}
6. Button Bersih :
private void btnbersihActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
btngrpmkn.clearSelection();
chidangan.setSelectedIndex(0);
tharga.setText("0");
ckemasan.setSelectedIndex(0);
ttotal.setText("0");
tpot.setText("0");
ttotber.setText("0");
tbayar.setText("0");
tkembali.setText("0");
rbtnayam.requestFocus();
}
7. Button Keluar :
private void btnkeluarActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
int keluar=JOptionPane.showConfirmDialog(null,"Ingin Keluar?","Pertanyaan",JOptionPane.YES_NO_OPTION);
if(keluar==0){
System.exit(0);
}
else{
return;
}
}
---------------------------------------------------------------------------------------
Sekian.