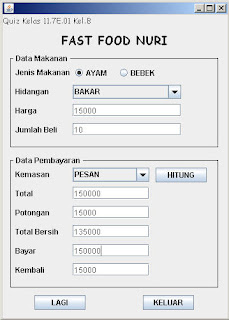
---------------------
1. Jenis Makanan :
- Ayam Goreng = Rp. 20.000,-
- Ayam Bakar = Rp. 15.000,-
- Bebek Goreng = Rp. 30.000,-
- Bebek Bakar = Rp. 25.000,-
2. Kemasan :
- Langsung = Diskon 50%
- Pesan = Diskon 10%
Source Code :
----------------
package mykalkulator;
import javax.swing.JOptionPane;
/**
*
* @author aie_sendiri
*/
public class QUIZ_FFOOD extends javax.swing.JFrame {
/** Creates new form QUIZ_FFOOD */
public QUIZ_FFOOD() {
initComponents();
}
1. Pembuatan Method :
@SuppressWarnings("unchecked")
Generated Code
void jenis(){
if(rbtnayam.isSelected() && chidangan.getSelectedItem().toString().equalsIgnoreCase("GORENG")){
txthrg.setText("20000");
txtbeli.requestFocus();
}
else if(rbtnayam.isSelected() && chidangan.getSelectedItem().toString().equalsIgnoreCase("BAKAR")){
txthrg.setText("15000");
txtbeli.requestFocus();
}
else if(rbtnbebek.isSelected() && chidangan.getSelectedItem().toString().equalsIgnoreCase("GORENG")){
txthrg.setText("30000");
txtbeli.requestFocus();
}
else if(rbtnbebek.isSelected() && chidangan.getSelectedItem().toString().equalsIgnoreCase("BAKAR")){
txthrg.setText("25000");
txtbeli.requestFocus();
}
else{
txthrg.setText("0");
}
}
2. Radio Button Ayam :
private void rbtnayamActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
jenis();
}
3. Radio Button Bebek :
private void rbtnbebekActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
jenis();
}
4. Combo Box Hidangan :
private void chidanganActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
jenis();
}
5. Button Hitung :
private void btnhitungActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
int hrg = Integer.parseInt(txthrg.getText());
int beli = Integer.parseInt(txtbeli.getText());
int total = hrg * beli;
txttotal.setText(String.valueOf(total));
if(ckemasan.getSelectedItem().toString().equalsIgnoreCase("LANGSUNG")){
int pot = total * 50/100;
int totber = total - pot;
txtpot.setText(String.valueOf(pot));
txttotber.setText(String.valueOf(totber));
txtbayar.requestFocus();
}
else if(ckemasan.getSelectedItem().toString().equalsIgnoreCase("PESAN")){
int pot = total * 10/100;
int totber = total - pot;
txtpot.setText(String.valueOf(pot));
txttotber.setText(String.valueOf(totber));
txtbayar.requestFocus();
}
else{
txtpot.setText("0");
txttotber.setText("0");
}
}
6. Text Field Bayar :
private void txtbayarActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
int bayar = Integer.parseInt(txtbayar.getText());
int totber = Integer.parseInt(txttotber.getText());
if(bayar>=totber){
int kembali = bayar - totber;
txtkembali.setText(String.valueOf(kembali));
}
else {
int kurang = JOptionPane.showConfirmDialog(null,"Uang Bayar Kurang !!","PERHATIAN",JOptionPane.OK_CANCEL_OPTION);
if(kurang == JOptionPane.OK_OPTION){
txtbayar.setText("");
txtkembali.setText("");
}
else{
txtbayar.setText("");
txtkembali.setText("");
}
}
}
7. Button Lagi :
private void btnlagiActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
btngrpmkn.clearSelection();
chidangan.setSelectedIndex(0);
txthrg.setText("");
txtbeli.setText("");
ckemasan.setSelectedIndex(0);
txttotal.setText("");
txtpot.setText("");
txttotber.setText("");
txtbayar.setText("");
txtkembali.setText("");
rbtnayam.requestFocus();
}
8. Button Keluar :
private void btnkeluarActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
int keluar = JOptionPane.showConfirmDialog(null,"Ingin Keluar ??","Pertanyaan",JOptionPane.YES_NO_OPTION);
if(keluar==JOptionPane.YES_OPTION){
System.exit(0);
}
else{
return;
}
}
Semoga Berguna !!