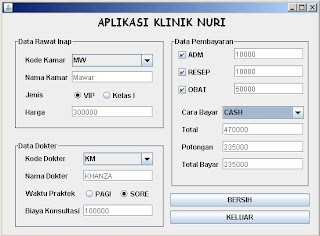
1. Kamar
---------------------------------
-Kode Kamar : BG
-Nama Kamar : Bougenville
---------------------------------
-Kode Kamar : MW
-Nama Kamar : Mawar
---------------------------------
2. Jenis Kamar
---------------------------------
-Jenis Kamar : VIP
-Harga Kamar : 300000
---------------------------------
-Jenis Kamar : Kelas I
-Harga Kamar : 200000
---------------------------------
3. Kode Dokter
---------------------------------
-Kode Dokter : KM
-Nama Dokter : Khanza
---------------------------------
-Kode Dokter : SA
-Nama Dokter : Syahniah
---------------------------------
4. Waktu Praktek
---------------------------------
-Waktu Praktek : PAGI
-Biaya Konsultasi : 50000
---------------------------------
-Waktu Praktek : SORE
-Biaya Konsultasi : 100000
---------------------------------
5. Biaya Lainnya
---------------------------------
-Admin : 10000
-Resep : 10000
-Obat : 50000
---------------------------------
6. Cara Pembayaran
---------------------------------
-Cash : diskon 50%
-Transfer : diskon 10%
---------------------------------
Source Code :
import javax.swing.JOptionPane;
/**
*
* @author aie_sendiri
*/
public class FKLINIK_NURI extends javax.swing.JFrame {
int hrg_kamar, b_konsul, adm, resep, obat, xpot, totyar, total;
double diskon, pot;
/** Creates new form FKLINIK_NURI */
public FKLINIK_NURI() {
initComponents();
}
---------------------------------------------------------------------------------------
1. Combo Box Kode Kamar :
private void ckamarActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
if(ckamar.getSelectedItem().toString().equalsIgnoreCase("bg")){
tkamar.setText("Bougenville");
}
else{
tkamar.setText("Mawar");
}
}
2. Radio Button Type Kamar VIP :
private void rvipActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
if(rvip.isSelected()){
hrg_kamar = 300000;
thrg.setText(String.valueOf(hrg_kamar));
}
}
3. Radio Button Type Kamar Kelas I :
private void rkelasActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
if(rkelas.isSelected()){
hrg_kamar = 200000;
thrg.setText(String.valueOf(hrg_kamar));
}
}
4. Combo Box Kode Dokter :
private void cdokterActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
if(cdokter.getSelectedItem().toString().equalsIgnoreCase("km")){
tnmdok.setText("KHANZA");
}
else{
tnmdok.setText("SYAHNIAH");
}
}
5. Radio Button Waktu Praktek Pagi :
private void rpagiActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
if(rpagi.isSelected()){
b_konsul = 50000;
tkonsul.setText(String.valueOf(b_konsul));
}
}
6. Radio Button Waktu Praktek Sore :
private void rsoreActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
if(rsore.isSelected()){
b_konsul = 100000;
tkonsul.setText(String.valueOf(b_konsul));
}
}
7. Check Box ADM :
private void chkadmActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
if(chkadm.isSelected()){
adm = 10000;
tadm.setText(String.valueOf(adm));
}
else{
adm = 0;
tadm.setText(String.valueOf(adm));
}
}
8. Check Box Resep :
private void chkresepActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
if(chkresep.isSelected()){
resep = 10000;
tresep.setText(String.valueOf(resep));
}
else{
resep = 0;
tresep.setText(String.valueOf(resep));
}
}
9. Check Box Obat :
private void chkobatActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
if(chkobat.isSelected()){
obat = 50000;
tobat.setText(String.valueOf(obat));
}
else{
obat = 0;
tobat.setText(String.valueOf(obat));
}
}
10. Combo Box Cara Bayar :
private void cbayarActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
if(cbayar.getSelectedItem().toString().equalsIgnoreCase("cash")){
diskon = 0.5;
total = hrg_kamar + b_konsul + adm + resep + obat;
pot = total * diskon;
xpot = (int)pot;
totyar = total - xpot;
ttotal.setText(String.valueOf(total));
tpot.setText(String.valueOf(xpot));
ttotyar.setText(String.valueOf(totyar));
}
else{
diskon = 0.1;
total = hrg_kamar + b_konsul + adm + resep + obat;
pot = total * diskon;
xpot = (int)pot;
totyar = total - xpot;
ttotal.setText(String.valueOf(total));
tpot.setText(String.valueOf(xpot));
ttotyar.setText(String.valueOf(totyar));
}
}
11. Button Bersih :
private void btnbersihActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
hrg_kamar = 0;
b_konsul = 0;
adm = 0;
resep = 0;
obat = 0;
xpot = 0;
totyar = 0;
total = 0;
diskon = 0;
pot = 0;
ckamar.setSelectedIndex(0);
cdokter.setSelectedIndex(0);
cbayar.setSelectedIndex(0);
btngrpkamar.clearSelection();
btngrpdokter.clearSelection();
tkamar.setText("");
thrg.setText("0");
tnmdok.setText("");
tkonsul.setText("0");
chkadm.setSelected(false);
chkresep.setSelected(false);
chkobat.setSelected(false);
tadm.setText("0");
tresep.setText("0");
tobat.setText("0");
ttotal.setText("0");
tpot.setText("0");
ttotyar.setText("0");
}
12. Button Keluar :
private void btnkeluarActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
int answer = JOptionPane.showConfirmDialog(null,"Ingin Keluar?","Pertanyaan",JOptionPane.YES_NO_OPTION);
if(answer == JOptionPane.YES_OPTION){
System.exit(0);
}
else{
return;
}
}
Semoga Bermanfaat !!
1 comment:
thanks for share, makin sukses
Post a Comment